
Preserve Encrypt Protect
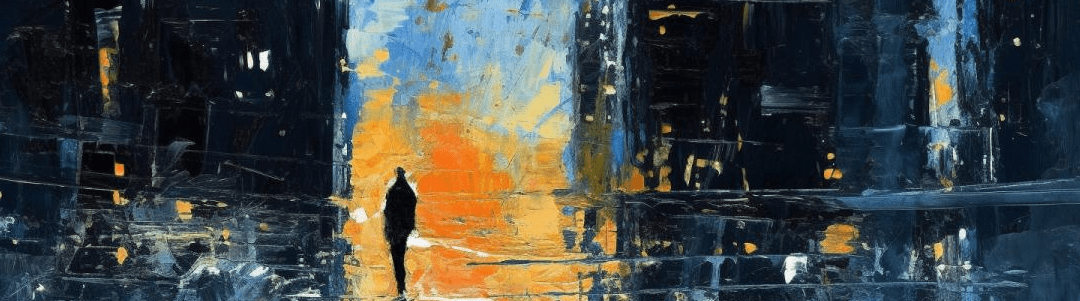
- Octomim provides a range of cutting-edge features designed to protect your valuable data and ensure its privacy.
- Octomim is a web-based RESTful FPE (Format-Preserving Encryption) application that goes above and beyond in safeguarding your sensitive information. With Octomim, you can preserve the integrity and structure of your data while keeping it encrypted and secure.
Key Features
- NIST FF3-1 Compliance: Octomim adheres to the NIST FF3-1 (Format-Preserving Encryption) standard. This compliance ensures that our encryption methods meet industry-recognized standards for data security and integrity.
Secured Secret Database: Octomim employs a secure secret database to store your sensitive information. Rest assured, your data is protected with robust encryption techniques, ensuring only authorized access.
Random Temporary Token Generation: As an added layer of security, Octomim generates random temporary tokens. These tokens provide secure access to your data for a limited time, adding an extra level of protection against unauthorized access.
Encrypted In-Memory Key Value Store: With our latest feature, Octomim offers an encrypted in-memory key-value store. This feature allows you to securely store and access key-value pairs in real-time, providing efficient and protected data retrieval.
- Steganographic Token: Hide your tokens in images secured with your key.
- Gatekeeper: Authenticate with multiple users. Create a gate with authorized users and set threshold of required number of users to open the gate.
- Gated Key Value Store: KV access is controlled via a gate. KV is attached to a gate that is administered by the Gatekeeper. If gate is open KV can be accessed, otherwise access is denied.
- Stateless. Decrypted data are not cached and they are securely deleted.
- Implemented in C for a blazing-fast performance.
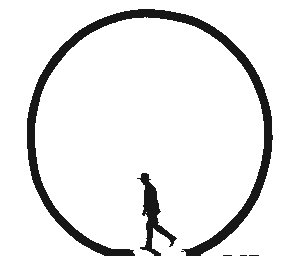
Encrypt
# Encrypt
curl -X POST -H "Content-Type: application/json" -d "{\"message\":\"mydata\"}" -u "USER:TOKEN" -k https://SERVER:PORT/api/encrypt
> {"result":"......"}
# Encrypt with salt
curl -X POST -H "Content-Type: application/json" -d "{\"message\":\"mydata\",\"salt\":\"mysalt\"}" -u "USER:TOKEN" -k https://SERVER:PORT/api/encrypt/salt
> {"result":"......"}
from octomim import Octomim
octo = Octomim(SERVER, PORT, USER, TOKEN)
response = octo.encrypt("ğüşıöç")
print("Encrypted:", response["result"])
response = octo.encrypt("mydata", salt="mysalt")
print("Encrypted with salt:", response["result"])
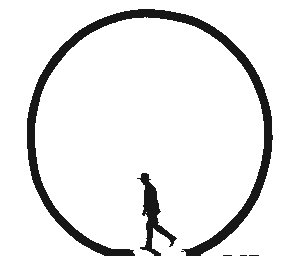
Decrypt
# Decrypt
curl -X POST -H "Content-Type: application/json" -d "{\"message\":\"......\"}" -u "USER:TOKEN" -k https://SERVER:PORT/api/decrypt
> {"result":"mydata"}
# Decrypt and mask
curl -X POST -H "Content-Type: application/json" -d "{\"message\":\"......\"}" -u "USER:TOKEN" -k https://SERVER:PORT/api/decrypt/mask
> {"result":"myd***"}
# Decrypt with salt
curl -X POST -H "Content-Type: application/json" -d "{\"message\":\"......\",\"salt\":\"mysalt\"}" -u "USER:TOKEN" -k https://SERVER:PORT/api/decrypt/salt
> {"result":"mydata"}
from octomim import Octomim
octo = Octomim(SERVER, PORT, USER, TOKEN)
response = octo.decrypt("JCWsğy")
print("Decrypted:", response["result"])
response = octo.decrypt("JCWsğy", mask=True)
print("Decrypted and masked:", response["result"])
response = octo.decrypt("PU0LuV", salt="mysalt")
print("Decrypted with salt",response["result"])
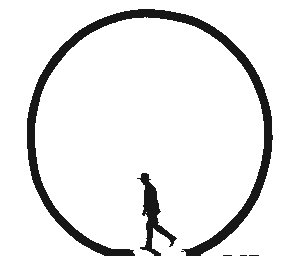
Bulk Encrypt
curl -s -X POST -H Content-Type: application/json -d {"messages":"data1;data2;data3;data4","salt":"NHB81YVbcj"} -u USER:TOKEN -k https://SERVER:PORT/api/encrypt/bulk
> { "messages": ".....;.....;.....;.....;" }
from octomim import Octomim
octo = Octomim(SERVER, PORT, USER, TOKEN)
# Encrypt bulk
response = octo.encrypt_bulk("data1;data2;data3")
print("Bulk Encrypted:", response["messages"])
# Encrypt bulk with salt
response = octo.encrypt_bulk("data1;data2;data3", salt="mysalt")
print("Bulk Encrypted with salt:", response["messages"])
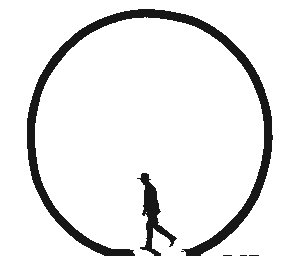
Bulk Decrypt
curl -s -X POST -H Content-Type: application/json -d {"messages":".....;.....;.....;.....;","salt":"NHB81YVbcj"} -u USER:TOKEN -k https://SERVER:PORT/api/decrypt/bulk
> { "messages": "data1;data2;data3;data4" }
from octomim import Octomim
octo = Octomim(SERVER, PORT, USER, TOKEN)
# Bulk decrypt
response = octo.decrypt_bulk("şöuv7;DrJlZ;8ĞVĞd")
print("Bulk Decrypted:", response["messages"])
# Bulk decrypt with salt
response = octo.decrypt_bulk("ejhUl;AYuGŞ;S2jİj", salt="mysalt")
print("Bulk Decrypted with salt:", response["messages"])
# Bulk decrypt and mask
response = octo.decrypt_bulk("şöuv7;DrJlZ;8ĞVĞd", mask=True)
print("Bulk Decrypted with salt and masked:", response["messages"])
# Bulk decrypt salt mask
response = octo.decrypt_bulk("ejhUl;AYuGŞ;S2jİj", mask=True, salt="mysalt")
print("Bulk Decrypted with salt and masked:", response["messages"])
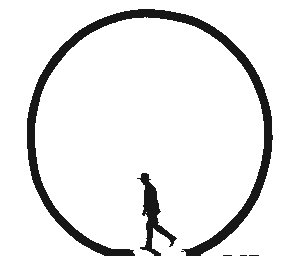
In-Memory Encrypted Key Value Store
# Set key-value
curl -X POST -H "Content-Type: application/json" -d "{\"key\":\"mykey\", \"value\":\"myvalue\"}" -u "USER:TOKEN" -k https://SERVER:PORT/api/ekv/set
> {"result":"ö72vvqO"} # This is stored in the memory and file
# Get value
curl -X POST -H "Content-Type: application/json" -d "{\"key\":\"mykey\"}" -u "USER:TOKEN" -k https://SERVER:PORT/api/ekv/get
> {"result":"myvalue"}
# Delete key-value
curl -X POST -H "Content-Type: application/json" -d "{\"key\":\"mykey\"}" -u "USER:TOKEN" -k https://SERVER:PORT/api/ekv/delete
> {"result":"Deleted"}
from octomim import Octomim
octo = Octomim(SERVER, PORT, USER, TOKEN)
response = octo.set_key_value("mykey", "ğüşiöç")
print("Set key-val:", response["result"])
response = octo.get_value("mykey")
print("Get value:", response["result"])
response = octo.delete_key_value("mykey")
print("Delete result:", response["result"])
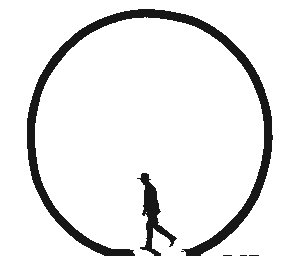
Token Generation
# Create temporary random token
curl -X POST -H "Content-Type: application/json" -d "{\"duration\":\"60\"}" -u "USER:TOKEN" -k https://SERVER:PORT/api/create/token
> {"token":"84DF44CD6C72707BD09C90CC01F31036CD675A84171CEE64982B6A3C4B965EAF"}
# Verify token
curl -X POST -H "Content-Type: application/json" -d "{\"token\":\"84DF44CD6C72707BD09C90CC01F31036CD675A84171CEE64982B6A3C4B965EAF\"}" -u "USER:TOKEN" -k https://SERVER:PORT/api/check/token
> {"result": "valid"}
# Verify Token
curl -X POST -H "Content-Type: application/json" -d "{\"token\":\"94DF44CD6C72707BD09C90CC01F31036CD675A84171CEE64982B6A3C4B965EAF\"}" -u "USER:TOKEN" -k https://SERVER:PORT/api/check/token
> {"error": "Token is invalid or expired"}
from octomim import Octomim
octo = Octomim(SERVER, PORT, USER, TOKEN)
response = octo.create_token("60") # duration 60 secs
print("Token:", response["token"])
response = octo.verify_token(response["token"])
print("Check token:", response["result"])
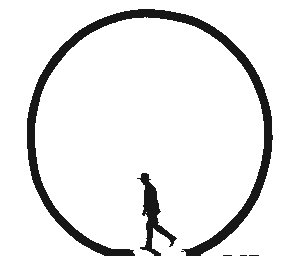
Gatekeeper
# Create gate
curl -X POST -H "Content-Type: application/json" -d "{\"gate_name\":\"Gate1\", \"users\":\"admin;octo;user3\", \"knock_threshold\":\"2\", \"gate_timeout\":\"10\", \"knock_timeout\":\"5\"}" -u "admin:TOKEN" -k https://SERVER:PORT/api/gate/create
> {"result":"Gate created"}
# Check gate
curl -X POST -H "Content-Type: application/json" -d "{\"gate_name\":\"Gate1\"}" -u "octo:TOKEN" -k https://SERVER:PORT/api/gate/check
> {"result":"Gate closed."}
# Knock
curl -X POST -H "Content-Type: application/json" -d "{\"gate_name\":\"Gate1\"}" -u "octo:TOKEN" -k https://SERVER:PORT/api/gate/knock
> {"result":"Gate knocked."}
# Knock
curl -X POST -H "Content-Type: application/json" -d "{\"gate_name\":\"Gate1\"}" -u "admin:TOKEN2" -k https://SERVER:PORT/api/gate/knock
> {"result":"Gate knocked."}
# Check
curl -X POST -H "Content-Type: application/json" -d "{\"gate_name\":\"Gate1\"}" -u "octo:TOKEN" -k https://SERVER:PORT/api/gate/check
> {"result":"Gate open."}
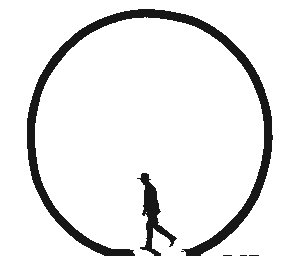
Steganographic Token Generation
# Stego: create token
curl -H "Content-Type: application/json" -u "USER:TOKEN" -k https://SERVER:PORT/api/stego/create/token
> {"file":"w26m7ms9aa.png", "token":"9002887DB2385BAA76BF5E46C3C7DAAF89963E4231FD9B72545574CB3BF8D607"}
# Stego: extract token
curl -X POST -H "Content-Type: application/json" -d "{\"file\":\"w26m7ms9aa.png\"}" -u "USER:TOKEN" -k https://SERVER:PORT/api/stego/extract/token
> {"token":"9002887DB2385BAA76BF5E46C3C7DAAF89963E4231FD9B72545574CB3BF8D607"}
# Get stego img
curl -X POST -H "Content-Type: application/json" -d "{\"file\":\"w26m7ms9aa.png\"}" -u "USER:TOKEN" -k https://SERVER:PORT/data/stego -o stego.png
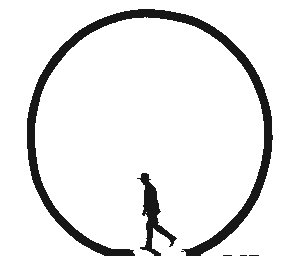
Gated Key Value Store
# Pre-created gate name
gate_name=Gate10
# Create Gated KV
curl -s -X POST -H "Content-Type: application/json" -d "{\"gate_name\":\"$gatename\", \"key\":\"$keyname\"}" -u "USER:TOKEN" -k "URL"
> Status returned
# Set GKV
curl -s -X POST -H "Content-Type: application/json" -d "{\"value\":\"$value\", \"key\":\"$keyname\"}" -u "USER:TOKEN" -k "URL"
> If gate is open value is set; otherwise fails.
# Get GKV
curl -s -X POST -H "Content-Type: application/json" -d "{\"key\":\"$keyname\"}" -u "USER:TOKEN" -k "URL"
> If gate is open value is received; otherwise fails.
# Delete GKV
curl -s -X POST -H "Content-Type: application/json" -d "{\"key\":\"$keyname\"}" -u "USER:TOKEN" -k "URL"
> Status returned
Seamless Integration with Databases via Octohook
SELECT name, iban FROM customers;
name |iban |
------------------+--------------------------+
Breanna Kreisler |XY023425588890426696346660|
Robert Earle |QR851687068551856211655866|
Mary Coleman |OI113123021027428390344254|
SELECT name, octohook(iban) FROM customers;
name |iban |
------------------+--------------------------+
Breanna Kreisler |TR533933332980106290102733|
Robert Earle |TR062098986102224590191780|
Mary Coleman |TR938114191340202605889213|
SELECT name, octohook(iban) as iban FROM people
HAVING iban LIKE 'TR5%';
name |iban |
------------------+--------------------------+
Breanna Kreisler |TR533933332980106290102733|
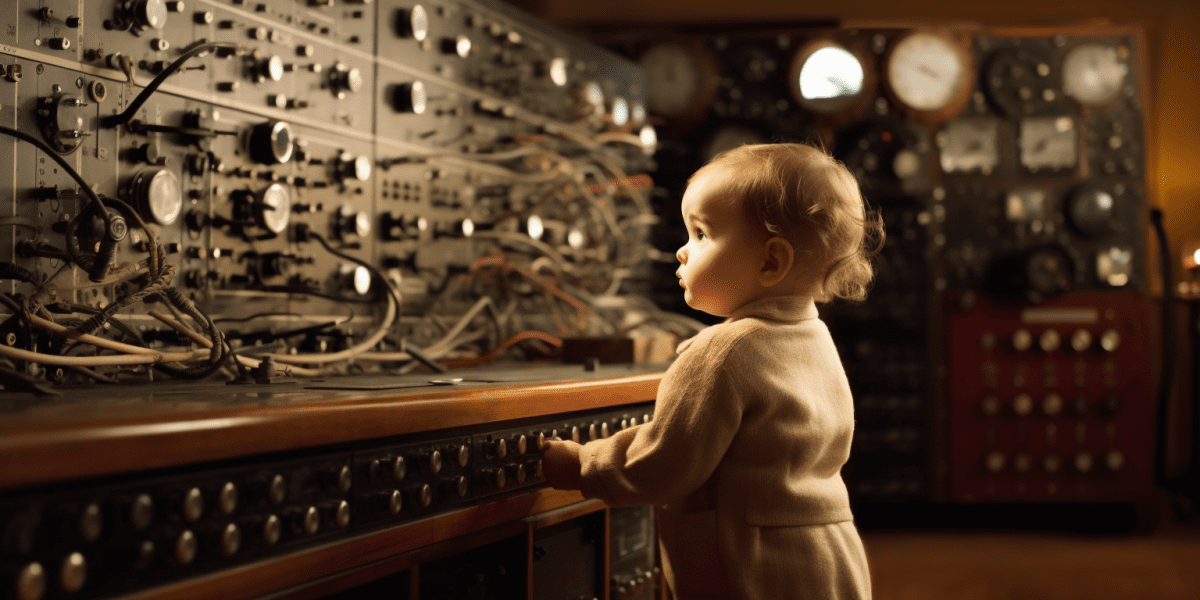
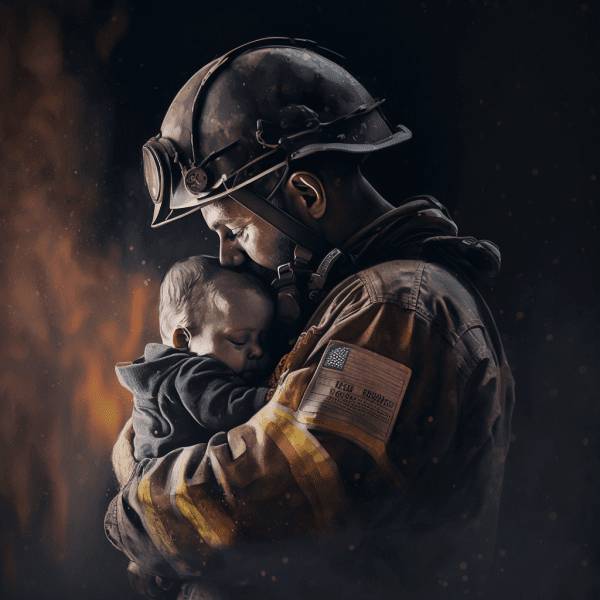
Don't compromise on the security of your data.
Choose Octomim - the web-based RESTful FPE solution that preserves, encrypts, and protects your valuable information.
Remember, with Octomim, your data is in safe hands.